We're happy to anounce that VisionX 5.1.221 is available!
It's a bigger update release of VisionX 5.1.8 with with some new "visible" features and many awesome new features under the hood.
Let's start with important bug fixes
- Popup Menu in Browser application
A custom Popup Menu in Browser applications was always empty. This problem was fixed in an OpenSource library and is now available in VisionX applications.
- Timeout with customizer changes
If VisionX timeout while a customizer with changed values was open, the timeout operation failed and VisionX was unusable.
- Element selection not always working
Sometimes it wasn't possible to select elements if they where behind other elements.
- Database procedure and function calls with more than 10 parameters
It's now supported to call database procedures and functions with max. 100 parameters.
- External CSS file detection
VisionX had some path problems with Linux and MacOS to find the external CSS file for browser applications.
- Fixed painting of overlays (MacOS)
The position of e.g. VisionX Guides wasn't correct. The small numbers were wrong positioned.
- Workflow integration
The workflow engine wasn't always started correctly and not in the correct window.
- All bugfixes of JVx, VaadinUI and other OpenSource libraries
What's new?
- A new Workflow AddOn
- Swagger documentation
The documentation now contains information about sub storages (used in drop down boxes) and default admin services. It's now easier to grant access to external applications based on the REST API of your application.
- Java 9+
It's now possible to use e.g. Java 11 as runtime for VisionX.
- Storage editor for Developers
Out storage Editor was only available if you bought the Forms license. Now all users with Development license will be able to use the Storage Editor. The storage editor is a feature which allows you to change the database query of a table, directly in VisionX.
- Prompt/Placeholder support
- Better layouting in browser application
We improved the layouting in our browser applications and also improved scrolling of panels.
- Experimental (Early Access) Features
We added some experimental features in this release: QR code authentication, Spnego (Active Directory) authentication base support (needs an additional AddOn), SSO authentication feature for REST services (bypass option).
This features are available for interested developers. Simply talk to your contact person.
The download area already contains links to latest VisionX binaries.
Please report any problems as usual and have fun with VisionX.
We're happy to announce that JVx 2.8 is available. It's a big release with lots of important bug fixes and some awesome new features. The last release of JVx was in January. It was version 2.7 which was more or less a small bugfix release with few new features. We took more time for JVx 2.8 to make it awesome.
What's new?
- Placeholder/Prompt support
This feature is available for input fields and cell editors. Simply enable this feature:
editor.setPlaceholderVisible(true);
editor.setPlaceholder("PLACEHOLDER");
to get

Prompt support
- New popup menu button
This new button reduces the visible components. It's a must have for modern applications:
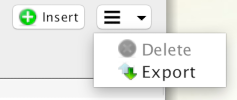
Menu button
- Savepoints for transaction handling in PostgreSql
- Components have a unique name
This is an important feature fore UI tests and our headless UI as well. It's now possible to address components in vaadin UI by their id to apply custom css.
- Configurable REST services
- Mac OS UI improvements
The full changelog is available here.
Start with JVx
Our VisionX solution store got a new demo application. It's a demo for calculated columns. A calculated column is a virtual column, filled with custom content.
Simply install the application from our solution store:

Demo application - Solution store
The application has 3 workscreens. One demonstrates client-side calculated columns, the second one server-side calculated columns and the third screen demonstrates calculated columns directly in the database. You have different options to use calculated columns, simply check what you prefer.
Our generic REST interface in JVx is well documented and different examples for different technologies are available. The generic approach itself is smart but not super specific because the implementation follows the CoC paradigm. It's awesome for "REST out-of-the-box" without additional coding effort, but the flexibility is sometimes missing.
We tried to break some of this restrictions and get more flexibility. Here is a list of new features to explain some details:
- Fetch on demand
The old implementation always returned all records from the database. It wasn't possible to implement paging or lazy loading. To solve this problem, we introduced new request parameters:
With _firstRow (starts from 0) you define the start record number. The _maxRows defines the amount of records for the resultset, e.g. start from 0 and return max. 20 records, start from 20 and return 20 records
- Configure visible columns
The old implementation returned the same column list for the REST call and the GUI/frontend. But sometimes the REST call should return a different list of columns because some internal columns aren't relevant or should be hidden. The new implementation got a new property in the metadata object: visibleColumnNames. If you set this property, the REST interface will return only the configure columns. The GUI won't recognize the new property because GUI controls have own visible column properties.
- Actions with Array parameters
The old implementation didn't support simple Arrays like BigDecimal[] as parameter types for action calls. Only List was supported. The new implementation supports Arrays but it's not possible to mix Array and List parameters. An example:
//valid
public String createPerson(String[] name, String[] title)
//valid
public String createPerson(List<String> name, List<String> title)
//invalid
public String createPerson(String[] name, List<String> title)
- New admin zone
We introduced a new admin zone for generic admin options. The URLs are:
http://.../services/rest/APPLICATION_NAME/_admin/ACTION_NAME
http://.../services/rest/APPLICATION_NAME/_admin/ACTION_NAME/ACTION_PARAM
Currently, we support following actions (POST requests): changePassword, testAuthentication
The request needs a JSON object with username, oldpassword, newpassword or password properties.
- Configurable zones
We now allow custom zones. The current default zones are:
http://.../services/rest/APPLICATION_NAME/_admin/...
http://.../services/rest/APPLICATION_NAME/LIFECYCLE_CLASS/data/OBJECT_NAME/...
http://.../services/rest/APPLICATION_NAME/LIFECYCLE_CLASS/action/ACTION_NAME/...
http://.../services/rest/APPLICATION_NAME/LIFECYCLE_CLASS/object/OBJECT_NAME/...
The zone names are: _admin, data, action, object
It's possible to set custom names with following parameters in the deployment descriptor: zone.admin, zone.data, zone.action, zone.object
- Hide object names
If you want to hide the object name(s), e.g.
https://.../demoerp/services/rest/DemoERP/Customers/data/customer/
The URL contains the Lifecycle Name (Customers) and the object name (customer). Both are easy to understand. In this example, we return the customer list from our customers object. If you want to use different names, it's possible to change the name in the lifecycle object code, e.g.
@Replacement(name="business")
public class Customers extends Session
{
@Replacement(name="clist")
public IStorage getCustomer()
{
...
}
}
With above code, it'll be possible to request:
https://.../demoerp/services/rest/DemoERP/business/data/clist/
The updated implementation is still a generic solution but it's now more flexible than before and should help you to offer new services. To use the new features, simply use our nightly JVx builds.
The support for ANT and JRE6 with Eclipse Photon.
The plugin was created for:
Version: Photon Release (4.8.0)
Build id: 20180619-1200
Don't forget the -clean start (read the original article for more details)!
One more thing you should do:
Photon includes ANT > 1.10. This version requires Java 8. In order to use Java 6, you have to download ANT 1.9.x. Simply download ANT and set the ANT Home to your version, via
(Window >>) Preferences >> ANT >> Runtime >> Ant Home...
If you try to use the bundled ANT with JRE 6, it will fail with an Exception.
Download the plugin from here. It works for us - no warranty!
More Details: Eclipse Oxygen.2 with ANT and JRE6.
We have some great Videos for new VisionX users on our YouTube channel.
Here are the links:
Getting started
How to create your first application
How to use Eclipse & VisionX in real time
Check them out!
These Videos will help new users learning VisionX
I'm happy to announce that our Workflow Engine will work with Oracle Forms.
The current state is beta but we'll release our Workflow engine in the next days. We found some time to test the integration in Oracle Forms and the result is soo cool. It works like a charm.
So it will be possible to embed our Workflow engine in your oracle Forms. It'll be possible for your users to design custom flows and processes. The engine itself is database driven and will work with your Oracle Forms application.
More details will follow in the next days, but here's a first impression:
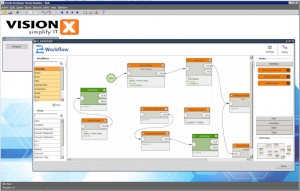
Workflow Engine - Oracle Forms
It's a standard Oracle Forms window/canvas with our Workflow Designer, embedded as Java Bean.
Feel free to ask your questions
We improved the support for standard MacOS LaF in our Swing UI and VisionX. The LaF has a lot of rendering problems. We tried to use another LaF implementation but had other problems. So we decided to keep the standard Java MacOS LaF.
The problems
- The InternalFrame border problem
- The field border problem
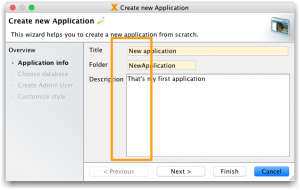 Field border problem |
A simple JTextField has a 5 pixel focus border. This is horrible because the focus is about 2 pixel. The other problem is that a JTextArea wrapped in a JScrollPane doesn't have the 5 pixel border. This makes it hard to create nice looking layouts.
We fixed this problem:
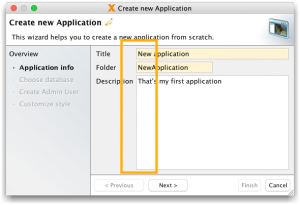 Field border without gap |
Looks better. One problem is that the JScrollPane doesn't paint the focus if the JTextArea has the focus. This is a LaF problem and we didn't find a workaround for this problem.
- Application Menubar
Standard Java appliacations show the menubar in the same frame
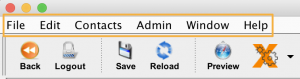 Application menu |
If you set the system property:
System.setProperty("apple.laf.useScreenMenuBar", "true");
the menu will be placed in the menu bar of MacOS:
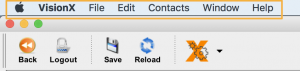 macOS Menu |
We fixed the problem that setting the menu to null will keep the menu as it was.
- Comboboxes
The height of Standard Comboboxes is wrong:
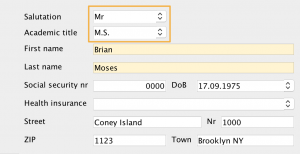 Combobox wrong |
We fixed the problem as good as possible:
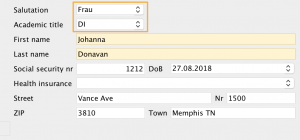 Combobox fixed |
- VisionX Toolbar height
The toolbar height was wrong because of layout calculation problems:
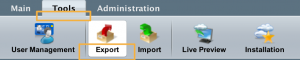 Wrong Toolbar height |
We fixed the problem and the toolbar height is correct:
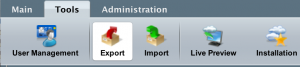 Toolbar fixed |
- VisionX Wizard gaps
The image gaps in VisionX wizards was not recognized:
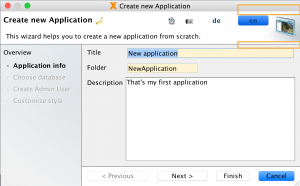 Wrong gaps |
It looks better without gaps:
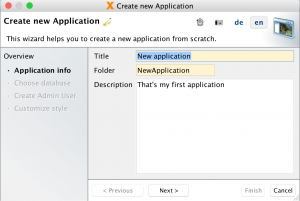 No gaps |
- Wrong button rendering
If you had a button without an image and a button with an image, the height of the button without image was higher than the button with an image. This is a LaF rendering bug:
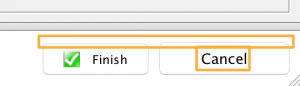 Wrong size and font |
The fonts are also different. We didn't know why there has to be such a difference, but we fixed the problem in Swing UI:
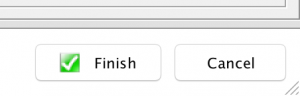 Button with correct size and font |
All changes are available in our nightly JVx builds
This article is a short introduction of our brand-new product. It's a super simple workflow engine. Our workflow engine is database driven and can be configured and extended for your needs. The engine itself contains a simple board which allows you to create workflows. The configuration will be read from and saved into a database. The workflow steps are configured in the database and the engine simply executes the configured steps.
We tried to find a workflow solution for our needs or a simple product which supports drawing workflows. We found a lot of complex workflow engines and tools but not a simple one. We didn't like complex solutions so we started with our own workflow enigne.
And here are some impressions
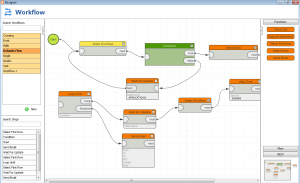 Workflow Designer (loop) |
|
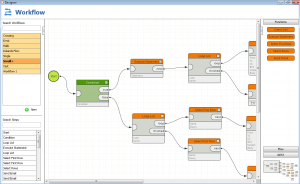 Workflow Designer |
The left area contains the list of available workflows and all used steps for each workflow. The search option is a full-text search for workflows and contained steps.
The right area contains all available/configured steps. The steps are configured in a database. We offer a simple Java interface which makes it possible to implement your own workflow steps. The area also contains a mini version of the board. This makes it smooth to search through the workflow.
The middle area, the board, contains the workflow designer. It supports Drag and Drop.
The created workflows can be used from any JVx based application. With next VisionX release, it'll be possible to use the new workflow engine.
The workflow engine will be available as AddOn for VisionX and as standalone version for product bundeling.
We're happy to anounce that VisionX 5.1.8 is available!
It's a simple update release of VisionX 5 with smaller bugfixes and one awesome new feature.
The bugs fixed
- Support for STARTS_WITH and CONTAINS Filter conditions
We didn't save this conditions correctly.
- Keyboard Navigation in HTML5 UI
It wasn't possible to use arrow keys for navigation in HTML5 tables.
- Floating License
We had some problems with floating license check with 1 user and the HTML5 live preview.
The new feature
The download area already contains links to VisionX 5.1 binaries.