JVx was started in 2008 as simple library for application development. The focus was on technology independce. It shouldn't matter which technology will be used in the future. In 2008, Swing was first choice for Java applications. In 2014, it will be JavaFX. JVx was designed to solve technology problems. It's not only a library, it's a full-stack-framework - an all in one solution for application development. But it's still a simple libray. There are no dependencies between the different application layers. Only use the persistence or an UI control or some utility classes. JVx is the right choice.
The big advantage of JVx is that it's API is simple and doesn't hide underlying technologies. Be technology independent or not - it's your decision!
The architecture is clean and easy to understand. The codebase is small and the source code meets the highest quality requirements. JVx is not only open source software, it's professional open source software.
What happened in the last few years with JVx?
We had some plans for JVx. The first was a core library for super-fast application development. This library is known as JVx. The other plans were about mobile applications, and different UI implementations like QT, GXT and a headless UI technique for automated tests. Of course, during recent years we played around with many libraries and frameworks like Pivot, GWT, vert.x, POI, ewsAPI, JasperReports, Birt, RAP, Jspresso, xdev, etc.
The result of our hard work is a framework that allows application development for desktop applications, web applications and mobile applications. BUT, the big difference to ALL other "hybrid" frameworks is that we write the application once and the same application runs without changes on ALL platforms.
JVx is a single-sourcing framework as well! Oh, and JVx is the only Java framework that allows a seamless integration in Oracle Forms.
We don't know a framework on this planet which is like JVx. It's small, it's simple, it's powerful, it's Open Source, it's professional, it's amazing.
Sounds unbelievable?
Maybe, but convince us that the opposite is true.
We know that JVx is not the solution for all problems because it was designed for database application development and not for other things. It's not a good idea to create a web-shop or a role-playing game with JVx. There are better tools for that. But if you develop backend applications that needs (or not) a modern web frontend and access with mobile devices, than JVx is your friend!
During the last few years, we had as much fun as possible. We put all our know how and time in JVx and we know that our vision will be reality! We show you two images that explains a little bit better what JVx covers
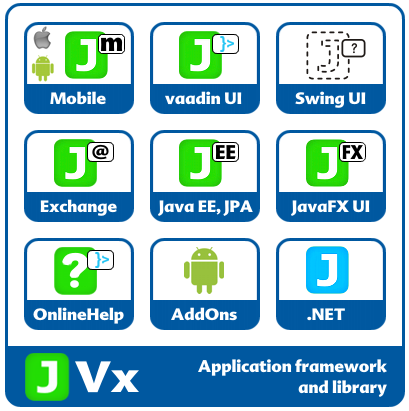
JVx' and independent projects
Above image shows projects built on-top of JVx. Following image shows what JVx - as library - supports. The list is not too detailed, but you should get an idea.
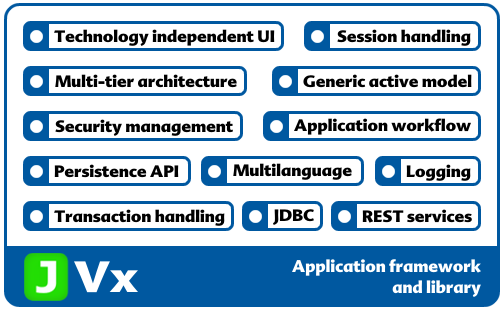
JVx' features
Our next steps?
The framework itself is great, but we need more documentation. We can't write as much howtos, intros as you need but we do our best. With JVx, we solve many problems but you don't know which ones without documentation. This is the biggest howto for 2013.
Another challenge is our JavaFX UI. We are working on it!
The next important milestone is the 1.0 Release of our vaadin UI. It's already code complete but we are still reviewing... be patient if you won't build on your own.